Promise vs Observable in Angular with (.subscribe, .then , .pipe) Best Explanations & Angular : BehaviorSubject
Part 01: Promise vs Observable in Angular with (.subscribe, .then , .pipe) Best Explanations
Observables & RxJS : video explanation
Observables
Streams so far are just a concept, an idea.
We link streams together using operators, so in in our previous example the add
function is an operation, specifically it’s an operation which combines two streams to create a third.
Observables is a new primitive type which acts as a blueprint for how we want to create streams, subscribe to them, react to new values, and combine streams together to build new ones.
It’s currently in discussion whether or not Observables make it into the ES7 version of JavaScript.
We are still trying to roll out ES6 so even if it makes it, it will be many years before ES7 becomes something we can code with natively.
Until then we need to use a library that gives us the Observable primitive and that’s where RxJS comes in.
RxJS
RxJS stands for *R*eactive E*x*tensions for *J*ava*S*cript, and it’s a library that gives us an implementation of Observables for JavaScript.
Note
RxJS is the JavaScript implementation of the ReactiveX API, which can be found here.
The API has multiple implementations in different languages, so if you learn RxJS you’ll know how to write RxJAVA, Rx.NET, RxPY etc…
Using RxJS with ES6
There are a couple of ways that you can add the RxJS library to your project.
With npm
you can use the following command:
Copynpm install rxjs
Alternately, you can also import RxJS from a CDN by including it in your HTML document:
Copy<script src="https://unpkg.com/rxjs/bundles/rxjs.umd.min.js"></script>
Note
The RxJS library also provides a number of Observable creation functions and operators (to build on the observables foundation) that can be added to your application via import statements like so:
Copyimport { map, filter, take } from "rxjs/operators";
import { interval, pipe } from "rxjs";
A Simple Example
Let’s understand the use of RxJS by working through a simple example.
Before we start adding any RxJS code, lets create a main.js
file with a barebones Angular implementation. This will hold our RxJS code; Specifically within the ngOnInit
function, a life cycle hook that is called once Angular is done creating the component.
Copyimport { NgModule, Component } from "@angular/core";
import { BrowserModule } from "@angular/platform-browser";
import { platformBrowserDynamic } from "@angular/platform-browser-dynamic";
@Component({
selector: "app",
template: `
<div>
<p>Observables & RxJS Example</p>
<p>(Please check the console)</p>
</div>
`
})
class AppComponent {
constructor() {}
ngOnInit() {
}
}
@NgModule({
imports: [BrowserModule],
declarations: [AppComponent],
bootstrap: [AppComponent]
})
class AppModule {}
platformBrowserDynamic().bootstrapModule(AppModule);
Next, we need to get an instance of an RxJS observable. There are numerous methods provided by the RxJS library for this, but for our example we will be using the interval
operator.
interval
An observable isn’t a stream. An observable is a blueprint which describes a set of streams and how they are connected together with operations
.
Lets assume for our use case, we want our observable to create a single stream and push onto that stream a number every second, incremented by 1.
For this purpose, we can use the interval
operator which creates an Observable that emits numbers in sequence based on the provided time interval like so:
Copyimport { interval } from 'rxjs';
const numbers = interval(1000);
The operation interval
takes as the first param the number of milliseconds between each push of the number onto the stream.
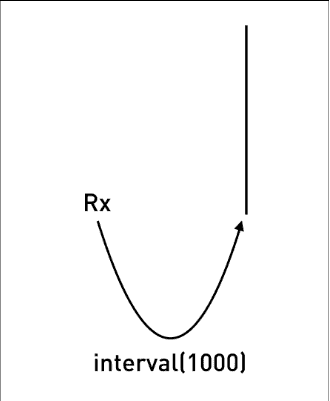
subscribe
Note
This observable is cold, that means it’s not currently pushing out numbers.
The observable will become hot and start pushing numbers onto it’s first stream, when it gets it’s first subscriber, like so:
Copyimport { interval } from 'rxjs';
const numbers = interval(1000);
numbers.subscribe(value => console.log("Subscriber: " + value));
By calling subscribe
onto an observable it:
Turns the observable hot so it starts producing.
Lets us pass in a callback function so we react when anything is pushed onto the final stream in the observable chain.
Our application now starts printing out:
CopySubscriber: 0 Subscriber: 1 Subscriber: 2 Subscriber: 3 Subscriber: 4 Subscriber: 5 Subscriber: 6 Subscriber: 7 Subscriber: 8 Subscriber: 9 Subscriber: 10
take
But it just keeps on printing, forever, we just want the first 3 items so we use another operator called take
.
We pass to that operator the number of items we want to take from the first stream. It creates a second stream and only pushes onto it the number of items we’ve requested, like so:
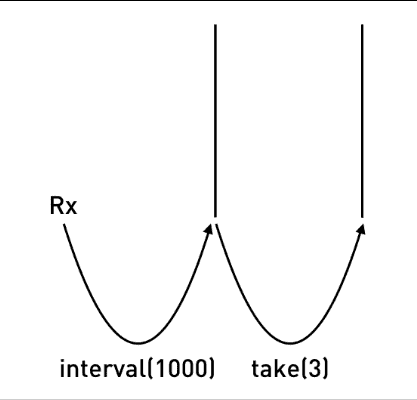
Copyimport { interval } from 'rxjs';
import { take } from 'rxjs/operators';
const numbers = interval(1000);
const takeThree = numbers.pipe(take(3));
takeThree.subscribe(value => console.log("Subscriber: " + value));
This now prints out the below, and then stops:
CopySubscriber: 0 Subscriber: 1 Subscriber: 2
Tip
pipe
is an Observable method which is used for composing operators. In more simpler terms, this allows us to chain operators together.map
Finally, I want to add another operator called map
, this takes as input the output stream from take
, convert each value to a date and pushes that out onto a third stream like so:
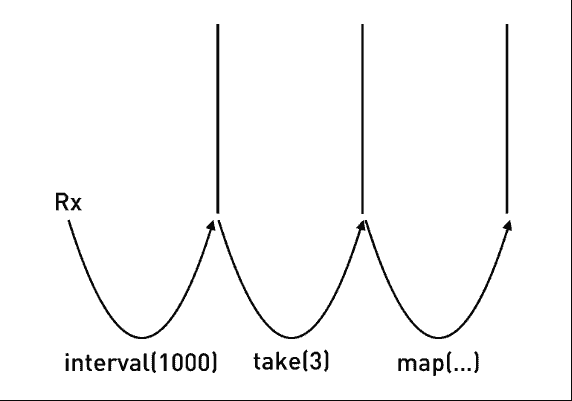
Copyimport { interval } from 'rxjs';
import { take, map } from 'rxjs/operators';
const numbers = interval(1000);
const takeThree = numbers.pipe(
take(3),
map((v) => Date.now())
);
takeThree.subscribe(value => console.log("Subscriber: " + value));
Note
pipe
to chain multiple operators together.This now prints out the time in milliseconds, every second, like so:
CopySubscriber: 1475506794287 Subscriber: 1475506795286 Subscriber: 1475506796285
Tip
Without declaring separate constants, we can also write the same code in a single statement like so:
Copyinterval(1000)
.pipe(
take(3),
map(v => Date.now())
)
.subscribe(value => console.log("Subscriber: " + value));
Other operators
The above example showed a very very small subset of the total number of operators available to you when using RxJS.
The hardest part of learning RxJS is understanding each of these operators and how to use them.
In that regard even though you are writing in JavaScript learning RxJS is closer to learning another language altogether.
You can find a list of the operators by looking at the official documentation here.
The documentation for the operators we just used above is:
Marble Diagrams
Trying to understand an operator by just reading some words is pretty difficult.
This is why in this lecture I’ve tried to use animations as much as possible.
The Rx team use something called a marble diagram to visually describe an operators function.
This is the official marble diagram for the map
operator:
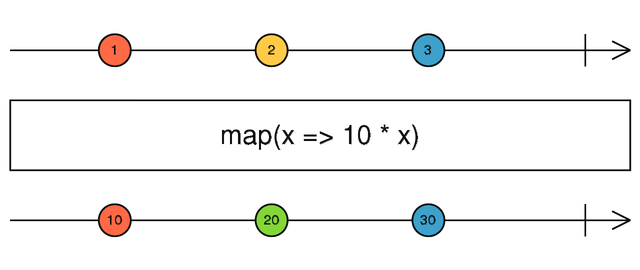
The line at the top represents time and the marbles with numbers 1, 2 and 3 represent the input stream over time.
The line at the bottom represents the output stream after each of the marbles has been processed through the operator.
The bit in the middle is the operator, in this example the operator is a
map
function which multiplies each marble in the input stream by 10 and pushes them to the output stream.
So in the above the value 1 gets pushed out onto the output stream as 10.
These diagrams are actually interactive.
To understand how an operator works we move the marbles around in the input stream and see how this affects the output stream, like so:
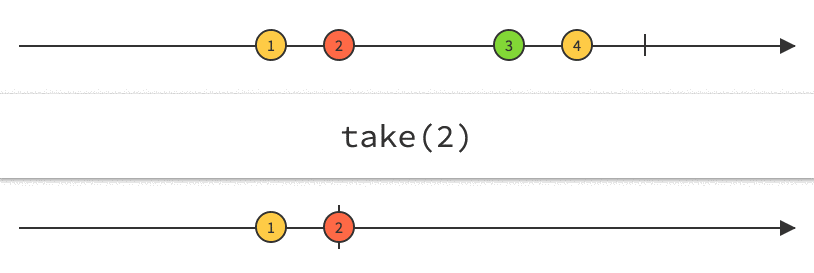
Summary
Observables are a blueprint for creating streams and plumbing them together with operators to create observable chains.
RxJS is a library that lets us create and work with observables.
We can subscribe to an observable chain and get a callback every time something is pushed onto the last stream.
By default observables are cold and only become hot when they get their first subscriber.
Learning RxJS involves understanding all the operators that are available and how to use them together.
We use marble diagrams to help explain how an operator works.
In this lecture we used RxJS in isolation, in the next lecture we will look at how to use RxJS in Angular.
Listing
Copyimport { NgModule, Component } from "@angular/core";
import { BrowserModule } from "@angular/platform-browser";
import { platformBrowserDynamic } from "@angular/platform-browser-dynamic";
import { map, filter, take } from "rxjs/operators";
import { interval, pipe } from "rxjs";
@Component({
selector: "app",
template: `
<div>
<p>Observables & RxJS Example</p>
<p>(Please check the console)</p>
</div>
`
})
class AppComponent {
constructor() {}
ngOnInit() {
interval(1000)
.pipe(
take(3),
map(v => Date.now())
)
.subscribe(value => console.log("Subscriber: " + value));
/*
const middleware = pipe(
take(3),
map(v => Date.now())
);
interval(1000)
.pipe(middleware)
.subscribe(value => console.log("Subscriber: " + value));
*/
}
}
@NgModule({
imports: [BrowserModule],
declarations: [AppComponent],
bootstrap: [AppComponent]
})
class AppModule {}
platformBrowserDynamic().bootstrapModule(AppModule);
Caught a mistake or want to contribute to the book? Edit this page on GitHub!
@@@@@@@@@@@ 2 nd Explanation @@@@@@@@@@@
################ 3 rd Explanation #################################### Advance best Explanation definitely Read below ########################################################################################
we will discuss the differences between promises and observables. In Angular 2, to work with asynchronous data we can use either Promises or Observables. In our previous videos in this series, we discussed using both Observables and Promises. There are several differences between Promises and Observables. In this video let's discuss these differences.
As a quick summary the differences are shown in the table below
Promise | Observable |
---|---|
Emits a single value | Emits multiple values over a period of time |
Not Lazy | Lazy. An Observable is not called until we subscribe to the Observable |
Cannot be cancelled | Can be cancelled using the unsubscribe() method |
Observable provides operators like map, forEach, filter, reduce, retry, retryWhen etc. |
A Promise emits a single value where as an Observable emits multiple values over a period of time. You can think of an Observable like a stream which emits multiple items over a period of time and the same callback function is called for each item emitted. So with an Observable we can use the same API to handle asynchronous data whether that data is emitted as a single value or multiple values over a period of time.
A Promise is not lazy where as an Observable is Lazy. Let's prove this with an example. Consider this method getEmployeeByCode() in employee.service.ts. Notice this method returns an Observable.
Here is the consumer code of the above service. In our example, this code is in employee.component.ts in ngOnInit() method. Notice we are subscribing to the Observable using the subscribe() method. An Observable is lazy because, it is not called and hence will not return any data until we subscribe using the subscribe() method. At the moment we are using the subscribe() method. So the service method should be called and we should receive data.
To prove this
1. Launch Browser developer tools by pressing F12 while you are on the browser.
2. Navigate to /src/employees/emp101
3. Click on the Network Tab
4. In the Filter textbox, type "api/employees"
5. At this point, you should only see the request issued to EmployeeService in the table below
6 Hover the mouse over "emp101" under "Name" column in the table

Instead of hovering over the request, if you click on it, you can see the response data as shown below. Make sure you select the "preview" tab.

Now in employee.component.ts file, comment the subscribe() method code block as shown below. Notice we are still calling the getEmployeeByCode() method of the EmployeeService. Since we have not subscribed to the Observable, a call to the EmployeeService will not be issued over the network.
With the above change in place, reload the web page. Notice no request is issued over the network to the EmployeeService. You can confirm this by looking at the network tab in the browser developer tools. So this proves that an Observable is lazy and won't be called unless we subscribe using the subscribe() method.
Now, let's prove that a Promise is NOT Lazy. I have modified the code in employee.service.ts to return a Promise instead of an Observable. The modified code is shown below.
Here is the consumer code of the above service. In our example, this code is in employee.component.ts in ngOnInit() method.
Because a promise is eager(not lazy), calling employeeService.getEmployeeByCode(empCode) will immediately fire off a request across the network to the EmployeeService. We can confirm this by looking at the network tab in the browser tools.
Now you may be thinking, then() method in this case is similar to subscribe() method. If we comment then() method code block, will the service still be called. The answer is YES. Since a Promise is NOT LAZY, Irrespective of whether you have then() method or not, calling employeeService.getEmployeeByCode(empCode) will immediately fire off a request across the network to the EmployeeService. You can prove this by commenting then() method code block and reissuing the request.
################################################################################################################################################
http://csharp-video-tutorials.blogspot.com/2017/09/angular-promises-vs-observables.htmlhttps://medium.com/@mpodlasin/promises-vs-observables-4c123c51fe13
Examples =: https://github.com/jpssasadara/web_Socket_Epic_POS_Final/tree/master/Fhttps://github.com/jpssasadara/Ex_Hub/tree/master/1.5%20Ex_hub
##################################################################################################################################################
Angular Observable pipe
pipe
example. RxJS pipe
is used to combine functional operators into a chain. pipe
is an instance method of Observable
as well as a standalone RxJS function. pipe
can be used as Observable.pipe
or we can use standalone pipe
to combine functional operators. The declaration of pipe
is as following.pipe
accepts operators as arguments such as filter
, map
, mergeScan
etc with comma separated and execute them in a sequence they are passed in as arguments and finally returns Observable
instance. To get the result we need to subscribe
it. Now let us discuss the complete example.echnologies Used
Find the technologies being used in our example.1. Angular 7.0.0
2. Angular CLI 7.0.3
3. TypeScript 3.1.1
4. Node.js 10.3.0
5. NPM 6.1.0
6. RxJS 6.3.3
7. In-Memory Web API 0.6.1
Import RxJS 6 Observable and Operators
In RxJS 6,Observable
and operators are imported as following.1. Import
Observable
and of
from rxjs
.pipe
will be accessed using Observable.pipe
. To use standalone pipe
, we can also import it.rxjs/operators
. Find some operators to import it.Observable.pipe with filter, map and scan
Here we will useObservable.pipe
to combine functions. Suppose we have a service method to get numbers.pipe
with filter
operator.We can observe that we are passing
filter
operator within pipe
. Final result of pipe
after executing filter
, will be instance of Observable
. To get the result we need to subscribe it.2. Find the code to use
pipe
with filter
and map
operators.In the above code we are passing
filter
and map
operators in pipe
function as arguments. filter
and map
will execute in the order they are passed in. On the Observable
instance returned by getNumbers()
, filter
operator will be executed and on the Observable
instance returned by filter
, map
operator will be executed and the final result returned by pipe
will be the result returned by last operator i.e. map
in above code.3. Find the code to use
pipe
with filter
, map
and scan
operators.In the above code we are using three operators with
pipe
i.e. filter
, map
and scan
. They will execute in the order they are passed in as arguments. In the above code, first filter
will execute then map
and then scan
. Final output returned by pipe
will be Observable
returned by scan
.Using Standalone pipe
To usepipe
standalone, we need to import it as following.Error Handling: pipe with retry and catchError
retry
operator reties to access URL for the given number of time before failing. catchError
has been introduced in RxJS 6. catchError
is used in place of catch
. Find the code to use pipe
with retry
and catchError
operator.Observable
instance.pipe with mergeMap
Find the code snippet to usepipe
with mergeMap
operator.pipe with switchMap
Find the code snippet to usepipe
with switchMap
operator.Complete Example
Here we will provide complete demo to usepipe
.book.component.ts
Run Application
To run the application, find the steps.1. Download source code using download link given below on this page.
2. Use downloaded src in your Angular CLI application. To install Angular CLI, find the link.
3. Install angular-in-memory-web-api@0.6.1
4. Run ng serve using command prompt.
5. Access the URL http://localhost:4200
Find the print screen of the output.

References
RxJS ObservableAngular: The RxJS library
Pipeable Operators
Download Source Code
Creating pipe ==> https://alligator.io/angular/custom-pipes-angular/
Comments
Post a Comment